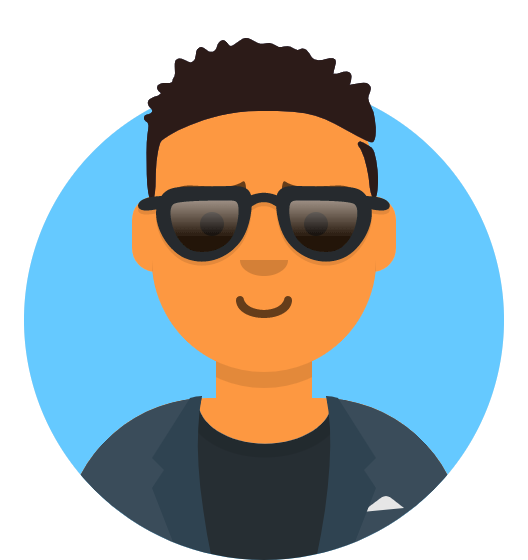
こんにちは!Masaです。
9回目は口座詳細ページの作成をしていきたいと思います。
- Django初心者で簡単なWebアプリケーション作成を考えている人
- Djangoの学び直しをしている人
- Djangoの基礎を身に付けたい人
前回のおさらいと今回やること
前回の記事では口座と収支の紐づけを行いました。
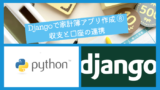
今回は、前回作成した資産詳細ページから各口座の詳しいページに移動できるようにしたいと思います。
各口座の詳細ページでは、利用履歴や残高推移を表示させます。
それでは頑張っていきましょう💪
各口座詳細ページの作成
各口座詳細ページの作成手順として
- 資産詳細ページで各口座を選択できるようにする
- 各口座の詳細ページを作成する
としたいと思います。
各口座を選択できるボタンを設置
まずはテンプレートを編集して、ボタンの設置をしましょう。
”by_account.html”を以下のように編集しましょう。
{% extends 'money_diary/base.html' %}
{% block content %}
<table class="table">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">口座</th>
<th scope="col">残高</th>
</tr>
</thead>
<tbody>
{% for item in account_balance %}
<tr>
<td><a type="button" class="btn btn-outline-primary" href="{% url 'account_detail' item.id %}" <!--追加-->選択</a></td>
<td>{{ item.name.name }}</td>
<td>{{ item.balance }}</td>
</tr>
{% endfor %}
</tbody>
</table>
{% endblock %}
選択ボタンを押すことでその口座の詳細ページに飛ぶことができます。
urls.pyへの追加
先ほどのテンプレートに新規のurlを記述したので、”urls.py”へ追加記述します。
アプリケーションファイル内(money_diary)の“urls.py”に以下のように追加します
from django.urls import path
from . import views
urlpatterns = [
path('', views.IndexView.as_view(), name='index'),
path('monthly_details/', views.Monthly_DetailsView.as_view(), name='monthly_details'),
path('monthly_details/<int:year>/<int:month>', views.Monthly_DetailsView.as_view(), name='monthly_details'),
path('by_account/', views.By_AccountView.as_view(), name='by_account'),
path('assets/', views.AssetsView.as_view(), name='assets'),
path('setup/', views.SetupView.as_view(), name='setup'),
path('income_delete/<int:num>', views.Income_DeleteView, name='income_delete'),
path('expense_delete/<int:num>', views.Expense_DeleteView, name='expense_delete'),
path('account_detail/<int:num>', views.Account_DetailView.as_view(), name='account_detail'), #追加
path('account_detail/<int:num>/<int:year>/<int:month>', views.Account_DetailView.as_view(), name='account_detail'), #追加
]
2つ追加しました。
やっていることは月別詳細ページを作成した時と同じですね。
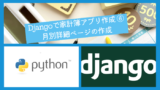
ビューの作成
次にビューの作成を行います。
こちらもやっていることはほとんど月別詳細ページと同じです。
“views.py”に以下のコードを追加記述してください。
class Account_DetailView(View):
def get(self, request, num, year=int(today[0]), month=int(today[1])):
# 選択口座のデータの取得
account = AccountBalance.objects.get(id=num)
# 選択講座の収支データの取得
income = Income.objects.all().filter(account__name=account.name, date__year=year, date__month=month).order_by()
expense = Expense.objects.all().filter(account__name=account.name, date__year=year, date__month=month).order_by()
# 月次切り替えの際の条件
if month == 12:
last_year = year
last_month = 11
next_year = year + 1
next_month = 1
elif month == 1:
last_year = year - 1
last_month = 12
next_year = year
next_month = 2
else:
last_year = year
last_month = month - 1
next_year = year
next_month = month + 1
params = {
'account': account,
'income': income,
'expense': expense,
'year': year,
'month': month,
'last_year': last_year,
'last_month': last_month,
'next_year': next_year,
'next_month': next_month,
}
return render(request, 'money_diary/account_detail.html', params)
収支データの取得では、「選択口座からの入出金データ&今月」という条件でfilter()を使用しています。
テンプレートの作成
最後に各口座の詳細が表示されるテンプレートを作成したいと思います。
各テンプレート(index.htmlなど)が入っているフォルダに”account_detail.html”を新規追加し、以下のコードを記述しましょう。
{% extends 'money_diary/base.html' %}
{% block content %}
<!-- 口座名と残高 -->
<h2>{{ account.name }}</h2>
<p>残高:{{ account.balance }}円</p>
<!-- 残高推移グラフ(後日作成) -->
<h3>残高推移</h3>
<!-- 年月の表示と前後の月への移動 -->
<nav aria-label="Page navigation example">
<ul class="pagination">
<li class="page-item">
<a class="page-link" href="{% url 'account_detail' account.id last_year last_month %}" aria-label="Previous">
<span aria-hidden="true">«</span>
</a>
</li>
<h1>{{ year }}年{{ month }}月</h1>
<li class="page-item">
<a class="page-link" href="{% url 'account_detail' account.id next_year next_month %}" aria-label="Next">
<span aria-hidden="true">»</span>
</a>
</li>
</ul>
</nav>
<!-- 収入の詳細テーブル -->
<h3>収入詳細</h3>
<table class="table">
<thead>
<tr>
<th scope="col">日付</th>
<th scope="col">内容</th>
<th scope="col">金額</th>
<th scope="col">金融機関</th>
<th scope="col">メモ</th>
</tr>
</thead>
<tbody>
{% for item in income %}
<tr>
<td>{{ item.date }}</td>
<td>{{ item.category }}</td>
<td>{{ item.amount }}</td>
<td>{{ item.account }}</td>
<td>{{ item.memo }}</td>
</tr>
{% endfor %}
</tbody>
</table>
<!-- 支出の詳細テーブル -->
<h3>支出詳細</h3>
<table class="table">
<thead>
<tr>
<th scope="col">日付</th>
<th scope="col">内容</th>
<th scope="col">金額</th>
<th scope="col">金融機関</th>
<th scope="col">メモ</th>
</tr>
</thead>
<tbody>
{% for item in expense %}
<tr>
<td>{{ item.date }}</td>
<td>{{ item.category }}</td>
<td>{{ item.amount }}</td>
<td>{{ item.account }}</td>
<td>{{ item.memo }}</td>
{% endfor %}
</tbody>
</table>
{% endblock %}
以上で完了です!
資産詳細ページから各口座の詳細へ移動できれば問題ないと思います。
資産推移のグラフは次の記事で作成していきます。
まとめ
今回は口座の一覧ページから各口座の詳細が見れる機能を追加しました。
あちらこちらにリンクがあったりして少し複雑ですが、やっていることは今までとあまり変わらないので少しづつ慣れていきましょう!
次回は各口座の残高推移のグラフを作成していきたいと思います。
最後まで読んでいただきありがとうございます。
自己学習に役立てていただけたら嬉しく思います
またSNSやブログでシェアしていただくことや、誤植などをコメントで指摘いただけたら幸いです。
次の記事↓
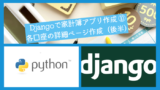
コメント